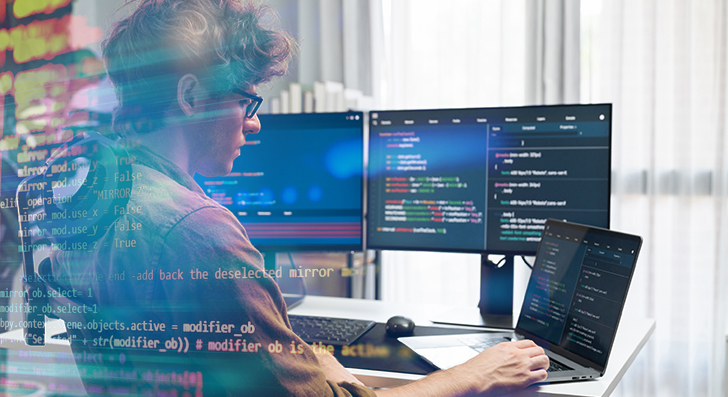
Scalability implies your application can manage development—much more buyers, additional knowledge, and a lot more site visitors—with out breaking. As being a developer, setting up with scalability in your mind saves time and worry later on. Right here’s a transparent and useful manual to help you get started by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability just isn't one thing you bolt on afterwards—it should be part within your plan from the start. Numerous purposes fail if they expand fast mainly because the original style and design can’t deal with the additional load. As being a developer, you'll want to Imagine early about how your process will behave under pressure.
Start off by designing your architecture to get adaptable. Stay away from monolithic codebases where by every little thing is tightly connected. As a substitute, use modular design or microservices. These patterns crack your app into more compact, unbiased parts. Each and every module or company can scale By itself without impacting The complete program.
Also, think of your database from working day one particular. Will it require to handle one million users or merely 100? Pick the correct kind—relational or NoSQL—determined by how your information will expand. System for sharding, indexing, and backups early, even if you don’t want them but.
A different significant place is to stay away from hardcoding assumptions. Don’t write code that only functions less than recent ailments. Take into consideration what would come about In the event your person base doubled tomorrow. Would your application crash? Would the database decelerate?
Use style and design styles that assistance scaling, like message queues or occasion-pushed programs. These assist your app take care of much more requests without the need of acquiring overloaded.
If you Construct with scalability in your mind, you are not just planning for achievement—you happen to be minimizing long term headaches. A perfectly-prepared technique is simpler to maintain, adapt, and mature. It’s superior to get ready early than to rebuild later on.
Use the appropriate Database
Selecting the right database is a vital Component of making scalable programs. Not all databases are constructed the same, and using the Completely wrong one can slow you down or simply lead to failures as your app grows.
Get started by knowledge your info. Can it be really structured, like rows in the table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. These are generally powerful with interactions, transactions, and consistency. In addition they guidance scaling strategies like read replicas, indexing, and partitioning to manage more website traffic and info.
In the event your knowledge is a lot more versatile—like person action logs, item catalogs, or paperwork—think about a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured facts and can scale horizontally a lot more conveniently.
Also, take into consideration your go through and produce patterns. Do you think you're doing a lot of reads with much less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Consider databases which will handle large publish throughput, or simply event-based mostly knowledge storage units like Apache Kafka (for temporary info streams).
It’s also smart to Feel forward. You might not will need advanced scaling attributes now, but selecting a database that supports them signifies you gained’t will need to modify later.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your details depending on your access patterns. And usually keep track of database overall performance as you improve.
Briefly, the ideal databases relies on your application’s framework, velocity desires, And just how you assume it to increase. Acquire time to select sensibly—it’ll help you save many issues later on.
Enhance Code and Queries
Quickly code is key to scalability. As your app grows, every compact hold off provides up. Inadequately prepared code or unoptimized queries can slow down efficiency and overload your method. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Avoid repeating logic and take away everything unneeded. Don’t select the most complex Alternative if an easy 1 works. Keep the functions shorter, focused, and simple to test. Use profiling applications to seek out bottlenecks—locations where your code can take as well extensive to run or uses an excessive amount memory.
Up coming, examine your databases queries. These usually gradual factors down more than the code by itself. Make certain Just about every query only asks for the info you actually need to have. Avoid SELECT *, which fetches almost everything, and rather pick out particular fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout large tables.
In case you see the identical data currently being asked for repeatedly, use caching. Retail outlet the results briefly applying tools like Redis or Memcached and that means you don’t really have to repeat costly operations.
Also, batch your databases operations once you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and helps make your app additional efficient.
Remember to check with substantial datasets. Code and queries that work good with 100 documents might crash once they have to deal with 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when needed. These steps aid your application continue to be smooth and responsive, whilst the load increases.
Leverage Load Balancing and Caching
As your application grows, it's got to handle additional end users plus more website traffic. If everything goes by a single server, it is going to quickly turn into a bottleneck. That’s in which load balancing and caching come in. Both of these resources support maintain your application quickly, secure, and scalable.
Load balancing spreads incoming site visitors across many servers. Rather than a person server undertaking many of the get the job done, the load balancer routes people to diverse servers depending on availability. This implies no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to setup.
Caching is about storing knowledge briefly so it might be reused rapidly. When users ask for the identical information yet again—like a product website page or perhaps a profile—you don’t should fetch it from the databases each and every time. You'll be able to provide it through the cache.
There are two prevalent varieties of caching:
one. Server-facet caching (like Redis or Memcached) merchants information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files near to the user.
Caching cuts down database load, increases speed, and tends to make your application much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when details does modify.
To put it briefly, load balancing and caching are straightforward but highly effective resources. Jointly, they assist your application deal with extra consumers, keep fast, and Recuperate from challenges. If you plan to expand, you require both.
Use Cloud and Container Equipment
To develop scalable applications, you will need instruments that let your app develop very easily. That’s the place cloud platforms and containers come in. They provide you adaptability, lessen set up time, and make scaling Considerably smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and companies as you require them. You don’t really have to invest in hardware or guess future capacity. When website traffic increases, you'll be able to incorporate far more methods with just a couple clicks or mechanically applying automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and safety equipment. You'll be able to target constructing your app rather than handling infrastructure.
Containers are An additional key Software. A container offers your app and all the things it ought to operate—code, libraries, settings—into 1 device. This can make it effortless to move your app concerning environments, from the laptop computer towards the cloud, without surprises. Docker is the preferred Resource for this.
Whenever your app employs numerous containers, applications like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and recovery. If 1 section of your respective app crashes, it restarts it quickly.
Containers also help it become simple to different areas of your app into expert services. You'll be able to update or scale parts independently, and that is great for overall performance and trustworthiness.
Briefly, making use of cloud and container applications indicates you can scale rapidly, deploy effortlessly, and Get well rapidly when challenges occur. If you prefer your app to improve with out boundaries, start employing these tools early. They preserve time, cut down possibility, and assist you to keep centered on creating, not correcting.
Monitor Almost everything
If you don’t monitor your application, you received’t know when things go Improper. Checking allows you see how your app is doing, location issues early, and make far better selections as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—observe your application too. Keep an eye on how long it will take for buyers to load pages, how website often errors happen, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Create alerts for crucial difficulties. By way of example, When your response time goes over a limit or perhaps a service goes down, you should get notified immediately. This helps you take care of challenges fast, often before buyers even detect.
Monitoring is additionally helpful when you make variations. When you deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge boost. Without checking, you’ll skip signs of trouble until eventually it’s also late. But with the right instruments in position, you continue to be in control.
In short, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it works very well, even under pressure.
Closing Thoughts
Scalability isn’t just for significant firms. Even small apps have to have a solid foundation. By planning cautiously, optimizing correctly, and using the proper applications, you'll be able to Establish apps that improve smoothly with no breaking stressed. Commence smaller, Believe massive, and Establish wise.